非单文件组件
文章发布较早,内容可能过时,阅读注意甄别。
# 基本使用
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>初识Vue</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<hello></hello>
<h2>{{msg}}</h2>
<school></school>
<student></student>
</div>
<div id="root2">
<hello></hello>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
// 第一步: 创建school组件
const school = Vue.extend({
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showName">点我提示学校名字</button>
</div>
`,
data() {
return {
schoolName: "尚硅谷",
address: "beijing",
};
},
methods: {
showName() {
alert(this.schoolName);
},
},
});
// 第一步: 创建student组件
const student = Vue.extend({
template: `
<div>
<h2>学生姓名:{{studentName}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
`,
data() {
return {
studentName: "张三",
age: 18,
};
},
methods: {
showName() {
alert(this.schoolName);
},
},
});
// 第一步: 创建hello组件
const hello = Vue.extend({
template: `
<div>
<h2>你好啊:{{name}}</h2>
</div>
`,
data() {
return {
name: "Tom",
};
},
});
// 第二步,全局注册组件
Vue.component("hello", hello);
new Vue({
el: "#root",
data: {
msg: "你好啊",
},
// 第二步,注册组件(局部注册)
components: {
school: school,
student: student,
},
});
new Vue({
el: "#root2",
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
# 笔记
- Vue 中使用组建的三个步骤:
- 定义组件(创建组件)
- 注册组件
- 使用组件(写组件标签)
- 如何定义一个组件?
使用
Vue.extend(options)
创建,其中 options 和new Vue(options)
时传入的那个 options 几乎一样,但也有点区别: 区别如下:
- el 不要写,为什么? 最终所有的组件都要经过一个 vm 的管理,由 vm 中的 el 决定服务哪个容器。
- data 必须写成函数式,为什么? 避免组件被复用时,数据存在引用关系。
备注:使用 template 可以配置组件结构。
如何注册组件?
局部注册:靠
new Vue
的时候传入components
选项全局注册:靠
Vue.components('组件名',组件)
编写组件标签:
<school></school>
# 几个注意点
# 代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>几个注意点</title>
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器-->
<div id="root">
<h1>{{msg}}</h1>
<school></school>
</div>
</body>
<script type="text/javascript">
Vue.config.productionTip = false;
//定义组件
const s = Vue.extend({
name: "atguigu",
template: `
<div>
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
`,
data() {
return {
name: "尚硅谷",
address: "北京",
};
},
});
new Vue({
el: "#root",
data: {
msg: "欢迎学习Vue!",
},
components: {
school: s,
},
});
</script>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
# 笔记
- 关于组件名:
- 一个单词组成:
- 第一种写法(首字母小写):school
- 第二种写法(首字母大写):School
- 多个单词组成:
- 第一种写法(kebab-case 命名):my-school
- 第二种写法(CamelCase 命名):MySchool (需要 Vue 脚手架支持)
- 备注:
- 组件名尽可能回避 HTML 中已有的元素名称,例如:h2、H2 都不行。
- 可以使用 name 配置项指定组件在开发者工具中呈现的名字。
- 关于组件标签:
- 第一种写法:
<school></school>
- 第二种写法:
<school/>
- 备注:不用使用脚手架时,
<school/>
会导致后续组件不能渲染。
- 一个简写方式:
const school = Vue.extend(options)
可简写为:const school = options
# 组件的嵌套
# 代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>几个注意点</title>
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器-->
<div id="root"></div>
</body>
<script type="text/javascript">
Vue.config.productionTip = false;
//定义组件
const student = Vue.extend({
template: `
<div>
<h2>学生姓名:{{name}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
`,
data() {
return {
name: "尚硅谷",
age: 18,
};
},
});
//定义组件
const school = Vue.extend({
template: `
<div>
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
<student></student>
</div>
`,
data() {
return {
name: "尚硅谷",
address: "北京",
};
},
components: {
student,
},
});
//定义组件
const hello = Vue.extend({
template: `
<div>
<h2>{{msg}}</h2>
</div>
`,
data() {
return {
msg: "欢迎来到尚硅谷学习!",
};
},
});
//定义组件
const app = Vue.extend({
template: `
<div>
<hello></hello>
<school></school>
</div>
`,
components: {
school,
hello,
},
});
new Vue({
el: "#root",
template: `<app></app>`,
// 注册组件(局部)
components: {
app,
},
});
</script>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
# VueComponent
# 代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>VueComponent</title>
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器-->
<div id="root">
<school></school>
<hello></hello>
</div>
</body>
<script type="text/javascript">
Vue.config.productionTip = false;
//定义组件
const school = Vue.extend({
template: `
<div>
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showName">点我提示学校名</button>
</div>
`,
data() {
return {
name: "尚硅谷",
address: "北京",
};
},
methods: {
showName() {
console.log("showName", this);
},
},
});
const test = Vue.extend({
template: `<span>eryajf</span>`,
});
//定义组件
const hello = Vue.extend({
template: `
<div>
<h2>{{msg}}</h2>
</div>
`,
data() {
return {
msg: "欢迎来到尚硅谷学习!",
};
},
components: { test },
});
console.log("@", school);
console.log("#", hello);
new Vue({
el: "#root",
// 注册组件(局部)
components: {
school,
hello,
},
});
</script>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
# 笔记
关于 VueComponent:
- school 组件本质是一个名为
VueComponent
的构造函数,且不是程序员定义的,是Vue.extend
生成的。 - 我们只需要写
<school/>
或<school></school>
,Vue 解析时会帮我们创建 school 组件的实例对象,即 Vue 帮我们执行的:new VueComponent(options)
。 - 特别注意:每次调用
Vue.extend
,返回的都是一个全新的 VueComponent
!!!! - 关于 this 指向:
组件
配置中:data
函数、methods
中的函数、watch
中的函数、computed
中的函数 它们的 this 均是VueComponent 实例对象
。new Vue(options)
配置中:data
函数、methods
中的函数、watch
中的函数、computed
中的函数 它们的 this 均是Vue 实例对象
。VueComponent
的实例对象,以后简称 vc(也可称之为:组件实例对象)。 Vue 的实例对象,以后简称 vm。
# 一个重要的内置关系
# 代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>组件-一个重要的内置关系</title>
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器-->
<div id="root">
<school></school>
<hello></hello>
</div>
</body>
<script type="text/javascript">
Vue.config.productionTip = false;
Vue.prototype.x = 99;
//定义组件
const school = Vue.extend({
template: `
<div>
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showX">点我提示学校名</button>
</div>
`,
data() {
return {
name: "尚硅谷",
address: "北京",
};
},
methods: {
showX() {
console.log("showName", this.x);
},
},
});
// 创建一个vm
const vm = new Vue({
el: "#root",
data: {
msg: "nihao",
},
components: { school },
});
</script>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
# 笔记
- 一个重要的内置关系:
VueComponent.prototype.__proto__ === Vue.prototype
- 为什么要有这个关系? 让组件实例对象(vc)可以访问到 Vue 原型上的属性,方法。
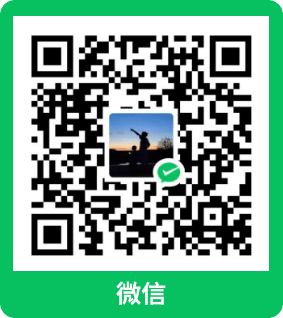
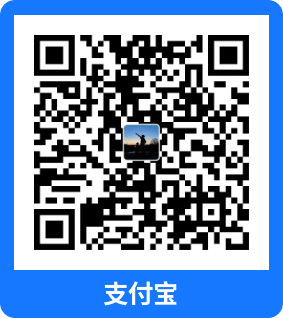
上次更新: 2024/11/19, 23:11:42