监视属性
文章发布较早,内容可能过时,阅读注意甄别。
# 天气案例
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>天气案例</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<h2>今天天气很{{info}}</h2>
<!-- 绑定事件的时候:@xxx="yyy" yyy可以写一些简单的语句 -->
<!-- <button @click="isHot = !isHot">切换天气</button> -->
<button @click="changeWeather">点我切换</button>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
new Vue({
el: "#root",
data: {
isHot: true,
},
computed: {
info() {
return this.isHot ? "炎热" : "凉爽";
},
},
methods: {
changeWeather() {
this.isHot = !this.isHot;
},
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
# 天气案例-监视属性
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>天气案例-监视属性</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<h2>今天天气很{{info}}</h2>
<button @click="changeWeather">点我切换</button>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
const vm = new Vue({
el: "#root",
data: {
isHot: true,
},
computed: {
info() {
return this.isHot ? "炎热" : "凉爽";
},
},
methods: {
changeWeather() {
this.isHot = !this.isHot;
},
},
// watch:{
// isHot:{
// immediate:true, // 初始化时让handler调用一下
// // 当isHot发生改变时,handler会被调用
// handler(newValue,oldValue){
// console.log('isHot被修改了',newValue,oldValue);
// }
// }
// }
});
vm.$watch("isHot", {
immediate: true, // 初始化时让handler调用一下
// 当isHot发生改变时,handler会被调用
handler(newValue, oldValue) {
console.log("isHot被修改了", newValue, oldValue);
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
# 笔记
监视属性 watch:
- 当被监视的属性变化时, 回调函数自动调用, 进行相关操作
- 监视的属性必须存在,才能进行监视!!
- 监视的两种写法:
new Vue
时传入 watch 配置- 通过
vm.$watch
监视
# 天气案例-深度监视
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>天气案例-监视属性</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!--
深度监视:
(1).Vue中的watch默认不监测对象内部值的改变(一层)。
(2).配置deep:true可以监测对象内部值改变(多层)。
备注:
(1).Vue自身可以监测对象内部值的改变,但Vue提供的watch默认不可以!
(2).使用watch时根据数据的具体结构,决定是否采用深度监视。
-->
<!-- 准备好一个容器 -->
<div id="root">
<h2>今天天气很{{info}}</h2>
<button @click="changeWeather">点我切换</button>
<hr />
<h3>a的值是:{{num.a}}</h3>
<button @click="num.a++">点我让a+1</button>
<hr />
<h3>b的值是:{{num.b}}</h3>
<button @click="num.b++">点我让b+1</button>
{{num.c.d.e}}
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
const vm = new Vue({
el: "#root",
data: {
isHot: true,
num: {
a: 1,
b: 2,
c: {
d: {
e: 4,
},
},
},
},
computed: {
info() {
return this.isHot ? "炎热" : "凉爽";
},
},
methods: {
changeWeather() {
this.isHot = !this.isHot;
},
},
watch: {
isHot: {
immediate: true, // 初始化时让handler调用一下
// 当isHot发生改变时,handler会被调用
handler(newValue, oldValue) {
console.log("isHot被修改了", newValue, oldValue);
},
},
// 监视结构中某个属性的变化
"num.a": {
// 当isHot发生改变时,handler会被调用
handler(newValue, oldValue) {
console.log("a被修改了", newValue, oldValue);
},
},
// 监视多级结构中所有属性的变化
num: {
deep: true, // 自动遍历内部
handler() {
console.log("num改变了");
},
},
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
# 笔记
深度监视:
- Vue 中的 watch 默认不监测对象内部值的改变(
一层
)。 - 配置
deep: true
可以监测对象内部值改变(多层
)。
备注:
- Vue 自身可以监测对象内部值的改变,但 Vue 提供的 watch 默认不可以!
- 使用 watch 时根据数据的具体结构,决定是否采用深度监视。
# 天气案例-监视属性-简写
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>天气案例-监视属性</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<h2>今天天气很{{info}}</h2>
<button @click="changeWeather">点我切换</button>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
const vm = new Vue({
el: "#root",
data: {
isHot: true,
},
computed: {
info() {
return this.isHot ? "炎热" : "凉爽";
},
},
methods: {
changeWeather() {
this.isHot = !this.isHot;
},
},
watch: {
// 正常写法
isHot: {
immediate: true, // 初始化时让handler调用一下
// 当isHot发生改变时,handler会被调用
handler(newValue, oldValue) {
console.log("isHot被修改了", newValue, oldValue);
},
},
// 简写 当监听的对象中只有handler参数的时候,可以如下简写
isHot(newValue, oldValue) {
console.log("isHot被修改了", newValue, oldValue);
},
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
# 姓名案例-watch 实现
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>姓名案例-watch实现</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
姓:<input type="text" v-model="firstName" /><br /><br />
名:<input type="text" v-model="lastName" /><br /><br />
全名:<span>{{fullName}}</span>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
new Vue({
el: "#root",
data: {
firstName: "张",
lastName: "三",
fullName: "张-三",
},
watch: {
firstName(val) {
setTimeout(() => {
console.log(this);
this.fullName = val + "-" + this.lastName;
}, 1000);
},
lastName(val) {
this.fullName = this.firstName + "-" + val;
},
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
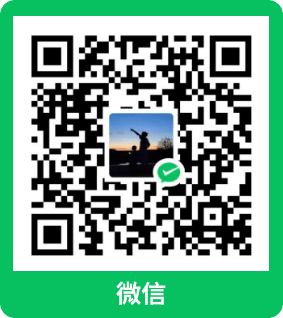
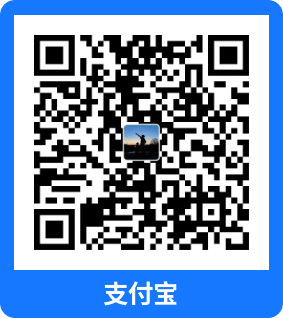
上次更新: 2025/02/20, 20:53:45